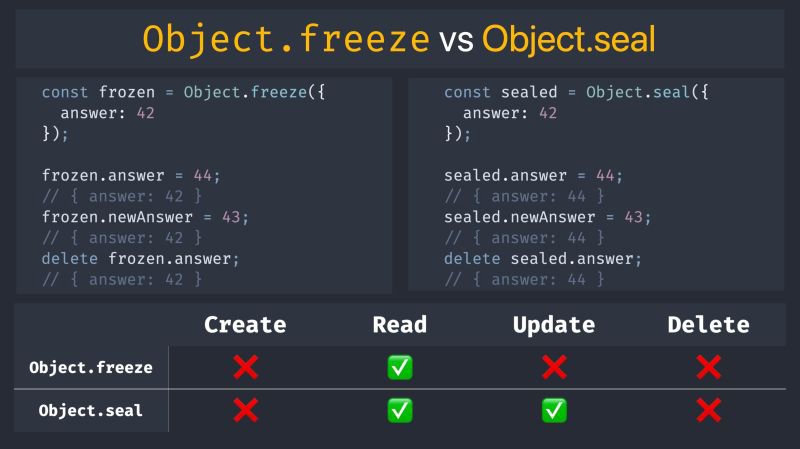
Object.Freeze and Object.seal
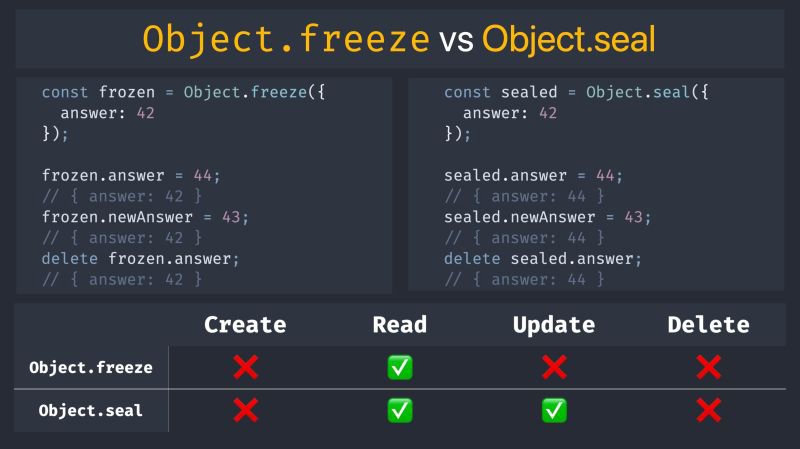
only for $ 9999
with all content and post
Contact Address:- ajaykumarui1234@gmail.com
1-Conditionally add a properties in object
const isValid = false
const age = 18
const person = {
id:'as32',
name:'ajay',
...(isValid && {isActive:true}), // (false && {isActive:true})
...((age>=18 || isValid) && {cart:0}) // (true && {cart:0})
}
console.log('person', person) // {id:as32, name:'ajay',cart:0}ly add a properties in object
j
2-Check a property exists in an object or not
const person = {
id:'as32',
name:'ajay',
}
console.log('name' in person) //true
console.log('isactive' in person) //false
3-Object destructing with dynamic key
const productData = {id:'23', name:'Dell'}
const {name:devicename} = productData
const extractKey = 'name'
const {[extractKey]:data} = productData
console.log('Data', data) // Data Dell
import { ActionTypes } from "../constants/action-types";
export const setProducts = (products) => {
const responser = await axios.get('/product')
return {
type: ActionTypes.SET_PRODUCTS,
payload: response,
};
};
export const selectedProduct = (product) => {
return {
type: ActionTypes.SELECTED_PRODUCT,
payload: product,
};
};
export const removeSelectedProduct = () => {
return {
type: ActionTypes.REMOVE_SELECTED_PRODUCT,
};
};
export const ActionTypes = {
SET_PRODUCTS: "SET_PRODUCTS",
SELECTED_PRODUCT: "SELECTED_PRODUCT",
REMOVE_SELECTED_PRODUCT: "REMOVE_SELECTED_PRODUCT",
};
import React, { useEffect } from "react";
import axios from "axios";
import { useParams } from "react-router-dom";
import { useDispatch, useSelector } from "react-redux";
import {
selectedProduct,
removeSelectedProduct,
} from "../redux/actions/productsActions";
const ProductDetails = () => {
const { productId } = useParams();
let product = useSelector((state) => state.product);
const { image, title, price, category, description } = product;
const dispatch = useDispatch();
const fetchProductDetail = async (id) => {
const response = await axios
.get(`https://fakestoreapi.com/products/${id}`)
.catch((err) => {
console.log("Err: ", err);
});
dispatch(selectedProduct(response.data));
};
useEffect(() => {
if (productId && productId !== "") fetchProductDetail(productId);
return () => {
dispatch(removeSelectedProduct());
};
}, [productId]);
return (
<div></div>
)}
Reusability — HOC
Small & Function-specific Components
Component Name After the Function
Component Names must be in Capitals
Lazy Loading
Use JSX ShortHand
Use Ternary Operators
Use Fragments
Don’t Define a Function Inside Render
Use Memo
Put CSS in JavaScript
Use Object Destructuring
Use Template Literals
//Ternary Operators
const { role } = user;
return role === ADMIN ? <AdminUser /> : <NormalUser />
const x = {
a: 1,
b: function() {
console.log(this);
}
}
x.b() /// {a: 1, b: ƒ}
const z = x.b // here this is window
z() // undefined
onst x = {
a: 1,
b: function() {
console.log(this);
}
}
x.b() /// {a: 1, b: ƒ}
const z = x.b // here this is window
z() // undefined
import react, { useState } from "react";
import { Switch, Route, Redirect} from "react-router-dom";
const App = ()=>{
return (
<Switch>
<Route path="/" exact component={Home} />
<Route path="/services" component={Service} />
</Switch>
)
}
export default App;
///////////////////////////////////////////////////////////////////
import {userouteWatch} from from "react-router-dom";
const {path, url} = userouteWatch ();
const Child= ()=>{
return (
<>
<Link to={`${url}/1234`} ></Link>
<Route path={`${path}/:id`} exact component={Home} />
<Route path="/services" component={Service} />
</>
)
}
export default Child
/////////////////////// Conditions ////
const Child= ()=>{
return (
<> <Route path={path} >
<Link to={`${url}/1234`} ></Link>
</Route>
<Route path={`${path}/:id`} exact component={Home} />
<Route path="/services" component={Service} />
</>
)
}
export default Child
import React, {usereducer} from 'react'
const initialstate = 0;
const reducer = (state, action)=>{
switch(action.type){
case 'increment':
return{
state+1
}
case: 'decrement':
retutn{
state-1
}
}
}
const MyComponent = ()=>{
const [state, dispatch] = useReducer(reducer, initialState);
return(
<>
{state}
<button onClick{()=> dispatch({type:'increment'})}>
increment
</button>
</>
)
}
Enums are one of the few features TypeScript has which is not a type-level extension of JavaScript. Enums allow a developer to define a set of named constants
enum Direction {
UP = 1,
DOWN,
LEFT,
RIGHT,
}
enum Role {
ADMIN:"Admin",
SUPERADMIN:"Super Admin",
}
enum Role {
NORMALUSER:"Normal User"
}
console.log(Role.ADMIN)
console.log(Role.ADMIN) // Admin
console.log(Role.NORMALUSER) // Normal User
Tuple
let person:[number,boolean, ...string]=[20, true, 'ajay']
“Profiler API” Its purpose is to help identify the part of an application that are slow so that we can check your component performance
So maybe benefit for optimizations
render(
<App>
<Profiler id="Panel" onRender={callback}>
<Panel {...props}>
<Profiler id="Content" onRender={callback}>
<Content {...props} />
</Profiler>
<Profiler id="PreviewPane" onRender={callback}>
<PreviewPane {...props} />
</Profiler>
</Panel>
</Profiler>
</App>
);
function onRenderCallback(
id, // the "id" prop of the Profiler tree that has just committed
phase, // either "mount" (if the tree just mounted) or "update" (if it re-rendered)
actualDuration, // time spent rendering the committed update
baseDuration, // estimated time to render the entire subtree without memoization
startTime, // when React began rendering this update
commitTime, // when React committed this update
interactions // the Set of interactions belonging to this update
) {
// Aggregate or log render timings...
}